Lớp FTPClient (org.apache.commons.net.ftp.FTPClient) của thư viện commons-net-3.3.jar cung cấp các API cần thiết để làm việc với một máy chủ thông qua giao thức FTP.
Phương thức FTPClient.retrieveFile() được sử dụng để download file from FTP Server.
Nội dung chính
Dưới đây là ví dụ về download file from FTP Server
Giả sử ta có list file và folder trong path="/demo" trên FTP Server như sau:
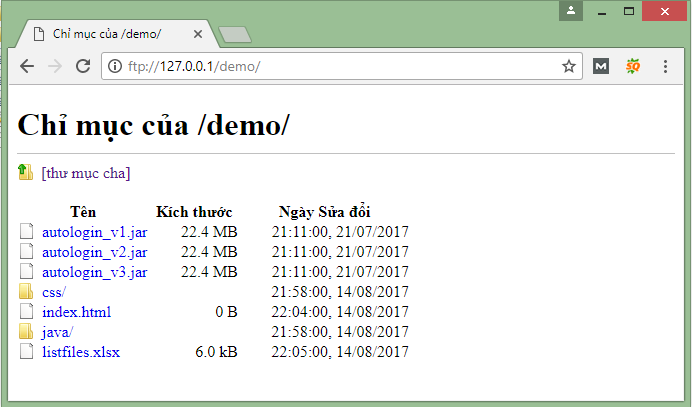
Chương trình sau sẽ download tất cả các file có phần mở rộng là ".jar" có trong path="/demo" trên FTP Server:
import java.io.BufferedOutputStream; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.io.OutputStream; import java.util.ArrayList; import java.util.List; import org.apache.commons.net.ftp.FTPClient; import org.apache.commons.net.ftp.FTPFile; import org.apache.commons.net.ftp.FTPFileFilter; import org.apache.commons.net.ftp.FTPReply; public class FTPExample { private static final String FTP_SERVER_ADDRESS = "127.0.0.1"; private static final int FTP_SERVER_PORT_NUMBER = 21; private static final int FTP_TIMEOUT = 60000; private static final int BUFFER_SIZE = 1024 * 1024 * 1; private static final String FTP_USERNAME = "haophong"; private static final String FTP_PASSWORD = "1234567890"; private static final String SLASH = "/"; private FTPClient ftpClient; /** * main * * @param args */ public static void main(String[] args) { String ftpFolder = "/demo"; String downloadFolder = "E:/ftpclient"; String extJarFile = "jar"; FTPExample ftpExample = new FTPExample(); // get list file ends with (.jar) from ftp server List<FTPFile> listFiles = ftpExample.getListFileFromFTPServer(ftpFolder, extJarFile); // download list file from ftp server and save to "E:/ftpclient" for (FTPFile ftpFile : listFiles) { ftpExample.downloadFTPFile(ftpFolder + SLASH + ftpFile.getName(), downloadFolder + SLASH + ftpFile.getName()); } } /** * using method retrieveFile(String, OutputStream) * to download file from FTP Server * * @author viettuts.vn * @param ftpFilePath * @param downloadFilePath */ private void downloadFTPFile(String ftpFilePath, String downloadFilePath) { System.out.println("File " + ftpFilePath + " is downloading..."); OutputStream outputStream = null; boolean success = false; try { File downloadFile = new File(downloadFilePath); outputStream = new BufferedOutputStream( new FileOutputStream(downloadFile)); // download file from FTP Server ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE); ftpClient.setBufferSize(BUFFER_SIZE); success = ftpClient.retrieveFile(ftpFilePath, outputStream); } catch (IOException e) { e.printStackTrace(); } finally { try { outputStream.close(); } catch (IOException e) { e.printStackTrace(); } } if (success) { System.out.println("File " + ftpFilePath + " has been downloaded successfully."); } } /** * get list from ftp server * * @author viettuts.vn * @param path * @return List<FTPFile> */ private List<FTPFile> getListFileFromFTPServer(String path, final String ext) { List<FTPFile> listFiles = new ArrayList<FTPFile>(); // connect ftp server connectFTPServer(); try { // list file ends with "jar" FTPFile[] ftpFiles = ftpClient.listFiles(path, new FTPFileFilter() { public boolean accept(FTPFile file) { return file.getName().endsWith(ext); } }); if (ftpFiles.length > 0) { for (FTPFile ftpFile : ftpFiles) { // add file to listFiles if (ftpFile.isFile()) { listFiles.add(ftpFile); } } } } catch (IOException ex) { ex.printStackTrace(); } return listFiles; } /** * connect ftp server * * @author viettuts.vn */ private void connectFTPServer() { ftpClient = new FTPClient(); try { System.out.println("connecting ftp server..."); // connect to ftp server ftpClient.setDefaultTimeout(FTP_TIMEOUT); ftpClient.connect(FTP_SERVER_ADDRESS, FTP_SERVER_PORT_NUMBER); // run the passive mode command ftpClient.enterLocalPassiveMode(); // check reply code if (!FTPReply.isPositiveCompletion(ftpClient.getReplyCode())) { disconnectFTPServer(); throw new IOException("FTP server not respond!"); } else { ftpClient.setSoTimeout(FTP_TIMEOUT); // login ftp server if (!ftpClient.login(FTP_USERNAME, FTP_PASSWORD)) { throw new IOException("Username or password is incorrect!"); } ftpClient.setDataTimeout(FTP_TIMEOUT); System.out.println("connected"); } } catch (IOException ex) { ex.printStackTrace(); } } /** * disconnect ftp server * * @author viettuts.vn */ private void disconnectFTPServer() { if (ftpClient != null && ftpClient.isConnected()) { try { ftpClient.logout(); ftpClient.disconnect(); } catch (IOException ex) { ex.printStackTrace(); } } } }
Kết quả:
connecting ftp server... connected File /demo/autologin_v1.jar is downloading... File /demo/autologin_v1.jar has been downloaded successfully. File /demo/autologin_v2.jar is downloading... File /demo/autologin_v2.jar has been downloaded successfully. File /demo/autologin_v3.jar is downloading... File /demo/autologin_v3.jar has been downloaded successfully.