Nội dung chính
- Bài tập quản lý sinh viên trong Java Swing
- Lời giải
- Tạo project qlsv-swing
- I. Tạo chức năng login
- 1. Tạo lớp User.java
- 2. Tạo lớp UserDao.java
- 3. Tạo lớp LoginView.java
- 4. Tạo lớp LoginController.java
- II. Tạo chức năng quản lý sinh viên
- 1. Tạo lớp Student.java
- 2. Tạo lớp StudentXML.java
- 3. Tạo lớp StudentDao.java
- 4. Tạo lớp StudentView.java
- 5. Tạo lớp StudentController.java
- 6. Tạo lớp FileUtils.java
- III. Tạo lớp App.java
- Run bài tập quản lý sinh viên trong java swing
- Download Project
Bài tập quản lý sinh viên trong Java Swing
Đề bài: Viết chương trình quản lý sinh viên trong Java , sử dụng Swing để tạo giao diện và áp dụng mô hình MVC. Mỗi đối tượng sinh viên có các thuộc tính sau: id, name, age, address và gpa (điểm trung bình). Với các chức năng sau:
- Sử dụng mô hình MVC.
- Tạo màn hình đăng nhập.
- Add student.
- Edit student.
- Delete student.
- Sắp xếp student theo GPA.
- Sắp xếp student theo Name.
- Hiển thị danh sách student.
- Lưu danh sách sinh viên vào file "student.xml".
Trong đó sinh viên được lưu vào file "student.xml" với định dạng xml. Ví dụ:
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <students> <student> <id>1</id> <name>Mai Thanh Toan</name> <age>22</age> <address>Ha Noi</address> <gpa>8.0</gpa> </student> <student> <id>2</id> <name>Vinh The Mac</name> <age>23</age> <address>Vinh Phuc</address> <gpa>9.5</gpa> </student> </students>
Lời giải
Chúng ta sẽ áp dụng mô hình MVC và Java Swing để tạo chương trình quản lý sinh viên.
Sử dụng maven để quản lý project, tham khảo bài tạo Maven project trong Eclipse .
MVC (viết tắt của Model-View-Controller) là một mẫu kiến trúc phần mềm hay mô hình thiết kế để tạo lập giao diện người dùng trên máy tính.
Đa số các dự án trong thực tế sử dụng mô hình MVC.
Tạo project qlsv-swing
Tạo maven project với cấu trúc của project trên eclipse:
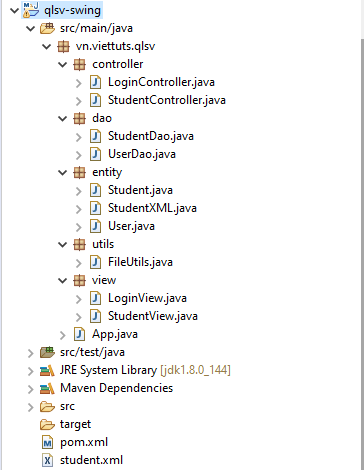
Tầng M (model) bao gồm package vn.viettuts.qlsv.dao và vn.viettuts.qlsv.entity
- Lớp User.java để lưu thông tin người dùng.
- Lớp UserDao.java chứa phương thức checkUser() để kiểm tra thông tin đang nhập.
- Lớp Student.java để lưu thông tin cho mỗi sinh viên.
- Lớp StudentXML.java để lưu thông tin danh sách sinh viên với định dạng XML vào file student.xml.
- Lớp StudentDao.java chứa các phương thức quản lý sinh viên như thêm, sửa, xóa, sắp xếp, đọc, ghi sinh viên.
Tầng V (view) bao gồm package vn.viettuts.qlsv.view
- Lớp LoginView.java tạo màn hình login.
- Lớp StudentView.java tạo màn hình quản lý sinh viên.
Tầng C (controller) bao gồm package vn.viettuts.qlsv.controller
- Lớp LoginController.java xử lý các sự kiện từ LoginView.java.
- Lớp StudentController.java xử lý các sự kiện từ StudentView.java.
Các file khác:
- Lớp FileUtils.java được sử dụng để đọc ghi file.
- Lớp App.java chứa hàm main để khởi chạy ứng dụng.
- File student.xml được sử dụng để lưu danh sách sinh viên.
Thêm các thư viện sau vào file pom.xml
- jaxb-api-2.3.0.jar : chuyển đối tượng thành xml và lưu vào file, đọc file và chuyển xml thành đối tượng.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>vn.viettuts</groupId> <artifactId>qlsv-swing</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>qlsv</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <!-- JAXB API --> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.3.1</version> </dependency> <!-- JAXB RI --> <dependency> <groupId>com.sun.xml.bind</groupId> <artifactId>jaxb-impl</artifactId> <version>2.3.4</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
I. Tạo chức năng login
Tạo màn hình login chứa thông tin sau:
- Trường username.
- Trường password.
- Login button.
1. Tạo lớp User.java
File: User.java
package vn.viettuts.qlsv.entity; public class User { private String userName; private String password; public User() { } public User(String userName, String password) { super(); this.userName = userName; this.password = password; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
2. Tạo lớp UserDao.java
File: UserDao.java
package vn.viettuts.qlsv.dao; import vn.viettuts.qlsv.entity.User; public class UserDao { public boolean checkUser(User user) { if (user != null) { if ("admin".equals(user.getUserName()) && "admin".equals(user.getPassword())) { return true; } } return false; } }
3. Tạo lớp LoginView.java
File: LoginView.java
package vn.viettuts.qlsv.view; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JPasswordField; import javax.swing.JTextField; import javax.swing.SpringLayout; import javax.swing.WindowConstants; import vn.viettuts.qlsv.entity.User; public class LoginView extends JFrame implements ActionListener { private static final long serialVersionUID = 1L; private JLabel userNameLabel; private JLabel passwordlabel; private JPasswordField passwordField; private JTextField userNameField; private JButton loginBtn; public LoginView() { initComponents(); } private void initComponents() { setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); userNameLabel = new JLabel("UserName"); passwordlabel = new JLabel("Password"); userNameField = new JTextField(15); passwordField = new JPasswordField(15); loginBtn = new JButton(); loginBtn.setText("Login"); loginBtn.addActionListener(this); // tạo spring layout SpringLayout layout = new SpringLayout(); JPanel panel = new JPanel(); // tạo đối tượng panel để chứa các thành phần của màn hình login panel.setSize(400, 300); panel.setLayout(layout); panel.add(userNameLabel); panel.add(passwordlabel); panel.add(userNameField); panel.add(passwordField); panel.add(loginBtn); // cài đặt vị trí các thành phần trên màn hình login layout.putConstraint(SpringLayout.WEST, userNameLabel, 20, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, userNameLabel, 80, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, passwordlabel, 20, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, passwordlabel, 105, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, userNameField, 80, SpringLayout.WEST, userNameLabel); layout.putConstraint(SpringLayout.NORTH, userNameField, 80, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, passwordField, 80, SpringLayout.WEST, passwordlabel); layout.putConstraint(SpringLayout.NORTH, passwordField, 105, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, loginBtn, 80, SpringLayout.WEST, passwordlabel); layout.putConstraint(SpringLayout.NORTH, loginBtn, 130, SpringLayout.NORTH, panel); // add panel tới JFrame this.add(panel); this.pack(); // cài đặt các thuộc tính cho JFrame this.setTitle("Login"); this.setSize(400, 300); this.setResizable(false); } public void showMessage(String message) { JOptionPane.showMessageDialog(this, message); } public User getUser() { return new User(userNameField.getText(), String.copyValueOf(passwordField.getPassword())); } public void actionPerformed(ActionEvent e) { } public void addLoginListener(ActionListener listener) { loginBtn.addActionListener(listener); } }
4. Tạo lớp LoginController.java
File: LoginController.java
package vn.viettuts.qlsv.controller; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import vn.viettuts.qlsv.dao.UserDao; import vn.viettuts.qlsv.entity.User; import vn.viettuts.qlsv.view.LoginView; import vn.viettuts.qlsv.view.StudentView; public class LoginController { private UserDao userDao; private LoginView loginView; private StudentView studentView; public LoginController(LoginView view) { this.loginView = view; this.userDao = new UserDao(); view.addLoginListener(new LoginListener()); } public void showLoginView() { loginView.setVisible(true); } /** * Lớp LoginListener * chứa cài đặt cho sự kiện click button "Login" * * @author viettuts.vn */ class LoginListener implements ActionListener { public void actionPerformed(ActionEvent e) { User user = loginView.getUser(); if (userDao.checkUser(user)) { // nếu đăng nhập thành công, mở màn hình quản lý sinh viên studentView = new StudentView(); StudentController studentController = new StudentController(studentView); studentController.showStudentView(); loginView.setVisible(false); } else { loginView.showMessage("username hoặc password không đúng."); } } } }
II. Tạo chức năng quản lý sinh viên
Tạo màn hình quản lý sinh viên chứa các thông tin sau:
- Các trường tương ứng với các thuộc tính của sinh viên.
- Button Add.
- Button Edit.
- Button Delete.
- Button Clear.
- Bảng hiển thị danh sách sinh viên.
- Button "Sort By Name"
- Button "Sort By GPA"
1. Tạo lớp Student.java
Lớp này để lưu thông tin cho mỗi sinh viên.
File: Student.java
package vn.viettuts.qlsv.entity; import java.io.Serializable; import javax.xml.bind.annotation.XmlAccessType; import javax.xml.bind.annotation.XmlAccessorType; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement(name = "student") @XmlAccessorType(XmlAccessType.FIELD) public class Student implements Serializable { private static final long serialVersionUID = 1L; private int id; private String name; private byte age; private String address; /* điểm trung bình của sinh viên */ private float gpa; public Student() { } public Student(int id, String name, byte age, String address, float gpa) { super(); this.id = id; this.name = name; this.age = age; this.address = address; this.gpa = gpa; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public byte getAge() { return age; } public void setAge(byte age) { this.age = age; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public float getGpa() { return gpa; } public void setGpa(float gpa) { this.gpa = gpa; } }
2. Tạo lớp StudentXML.java
File: StudentXML.java
package vn.viettuts.qlsv.entity; import java.util.List; import javax.xml.bind.annotation.XmlAccessType; import javax.xml.bind.annotation.XmlAccessorType; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement(name = "students") @XmlAccessorType(XmlAccessType.FIELD) public class StudentXML { private List<Student> student; public List<Student> getStudent() { return student; } public void setStudent(List<Student> student) { this.student = student; } }
3. Tạo lớp StudentDao.java
Tạo file "student.xml" tại thư mục gốc của dự án để lưu danh sách sinh viên.
File: StudentDao.java
package vn.viettuts.qlsv.dao; import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; import vn.viettuts.qlsv.entity.Student; import vn.viettuts.qlsv.entity.StudentXML; import vn.viettuts.qlsv.utils.FileUtils; /** * StudentDao class * * @author viettuts.vn */ public class StudentDao { private static final String STUDENT_FILE_NAME = "student.xml"; private List<Student> listStudents; public StudentDao() { this.listStudents = readListStudents(); if (listStudents == null) { listStudents = new ArrayList<Student>(); } } /** * Lưu các đối tượng student vào file student.xml * * @param students */ public void writeListStudents(List<Student> students) { StudentXML studentXML = new StudentXML(); studentXML.setStudent(students); FileUtils.writeXMLtoFile(STUDENT_FILE_NAME, studentXML); } /** * Đọc các đối tượng student từ file student.xml * * @return list student */ public List<Student> readListStudents() { List<Student> list = new ArrayList<Student>(); StudentXML studentXML = (StudentXML) FileUtils.readXMLFile( STUDENT_FILE_NAME, StudentXML.class); if (studentXML != null) { list = studentXML.getStudent(); } return list; } /** * thêm student vào listStudents và lưu listStudents vào file * * @param student */ public void add(Student student) { int id = 1; if (listStudents != null && listStudents.size() > 0) { id = listStudents.size() + 1; } student.setId(id); listStudents.add(student); writeListStudents(listStudents); } /** * cập nhật student vào listStudents và lưu listStudents vào file * * @param student */ public void edit(Student student) { int size = listStudents.size(); for (int i = 0; i < size; i++) { if (listStudents.get(i).getId() == student.getId()) { listStudents.get(i).setName(student.getName()); listStudents.get(i).setAge(student.getAge()); listStudents.get(i).setAddress(student.getAddress()); listStudents.get(i).setGpa(student.getGpa()); writeListStudents(listStudents); break; } } } /** * xóa student từ listStudents và lưu listStudents vào file * * @param student */ public boolean delete(Student student) { boolean isFound = false; int size = listStudents.size(); for (int i = 0; i < size; i++) { if (listStudents.get(i).getId() == student.getId()) { student = listStudents.get(i); isFound = true; break; } } if (isFound) { listStudents.remove(student); writeListStudents(listStudents); return true; } return false; } /** * sắp xếp danh sách student theo name theo tứ tự tăng dần */ public void sortStudentByName() { Collections.sort(listStudents, new Comparator<Student>() { public int compare(Student student1, Student student2) { return student1.getName().compareTo(student2.getName()); } }); } /** * sắp xếp danh sách student theo GPA theo tứ tự tăng dần */ public void sortStudentByGPA() { Collections.sort(listStudents, new Comparator<Student>() { public int compare(Student student1, Student student2) { if (student1.getGpa() > student2.getGpa()) { return 1; } return -1; } }); } public List<Student> getListStudents() { return listStudents; } public void setListStudents(List<Student> listStudents) { this.listStudents = listStudents; } }
4. Tạo lớp StudentView.java
File: StudentView.java
package vn.viettuts.qlsv.view; import java.awt.Dimension; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.List; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTable; import javax.swing.JTextArea; import javax.swing.JTextField; import javax.swing.SpringLayout; import javax.swing.WindowConstants; import javax.swing.event.ListSelectionEvent; import javax.swing.event.ListSelectionListener; import javax.swing.table.DefaultTableModel; import vn.viettuts.qlsv.entity.Student; public class StudentView extends JFrame implements ActionListener, ListSelectionListener { private static final long serialVersionUID = 1L; private JButton addStudentBtn; private JButton editStudentBtn; private JButton deleteStudentBtn; private JButton clearBtn; private JButton sortStudentGPABtn; private JButton sortStudentNameBtn; private JScrollPane jScrollPaneStudentTable; private JScrollPane jScrollPaneAddress; private JTable studentTable; private JLabel idLabel; private JLabel nameLabel; private JLabel ageLabel; private JLabel addressLabel; private JLabel gpaLabel; private JTextField idField; private JTextField nameField; private JTextField ageField; private JTextArea addressTA; private JTextField gpaField; // định nghĩa các cột của bảng student private String [] columnNames = new String [] { "ID", "Name", "Age", "Address", "GPA"}; // định nghĩa dữ liệu mặc định của bẳng student là rỗng private Object data = new Object [][] {}; public StudentView() { initComponents(); } private void initComponents() { setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); // khởi tạo các phím chức năng addStudentBtn = new JButton("Add"); editStudentBtn = new JButton("Edit"); deleteStudentBtn = new JButton("Delete"); clearBtn = new JButton("Clear"); sortStudentGPABtn = new JButton("Sort By GPA"); sortStudentNameBtn = new JButton("Sort By Name"); // khởi tạo bảng student jScrollPaneStudentTable = new JScrollPane(); studentTable = new JTable(); // khởi tạo các label idLabel = new JLabel("Id"); nameLabel = new JLabel("Name"); ageLabel = new JLabel("Age"); addressLabel = new JLabel("Address"); gpaLabel = new JLabel("GPA"); // khởi tạo các trường nhập dữ liệu cho student idField = new JTextField(6); idField.setEditable(false); nameField = new JTextField(15); ageField = new JTextField(6); addressTA = new JTextArea(); addressTA.setColumns(15); addressTA.setRows(5); jScrollPaneAddress = new JScrollPane(); jScrollPaneAddress.setViewportView(addressTA); gpaField = new JTextField(6); // cài đặt các cột và data cho bảng student studentTable.setModel(new DefaultTableModel((Object[][]) data, columnNames)); jScrollPaneStudentTable.setViewportView(studentTable); jScrollPaneStudentTable.setPreferredSize(new Dimension (480, 300)); // tạo spring layout SpringLayout layout = new SpringLayout(); // tạo đối tượng panel để chứa các thành phần của màn hình quản lý Student JPanel panel = new JPanel(); panel.setSize(800, 420); panel.setLayout(layout); panel.add(jScrollPaneStudentTable); panel.add(addStudentBtn); panel.add(editStudentBtn); panel.add(deleteStudentBtn); panel.add(clearBtn); panel.add(sortStudentGPABtn); panel.add(sortStudentNameBtn); panel.add(idLabel); panel.add(nameLabel); panel.add(ageLabel); panel.add(addressLabel); panel.add(gpaLabel); panel.add(idField); panel.add(nameField); panel.add(ageField); panel.add(jScrollPaneAddress); panel.add(gpaField); // cài đặt vị trí các thành phần trên màn hình login layout.putConstraint(SpringLayout.WEST, idLabel, 10, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, idLabel, 10, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, nameLabel, 10, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, nameLabel, 40, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, ageLabel, 10, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, ageLabel, 70, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, addressLabel, 10, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, addressLabel, 100, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, gpaLabel, 10, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, gpaLabel, 200, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, idField, 100, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, idField, 10, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, nameField, 100, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, nameField, 40, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, ageField, 100, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, ageField, 70, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, jScrollPaneAddress, 100, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, jScrollPaneAddress, 100, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, gpaField, 100, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, gpaField, 200, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, jScrollPaneStudentTable, 300, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, jScrollPaneStudentTable, 10, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, addStudentBtn, 20, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, addStudentBtn, 240, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, editStudentBtn, 60, SpringLayout.WEST, addStudentBtn); layout.putConstraint(SpringLayout.NORTH, editStudentBtn, 240, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, deleteStudentBtn, 60, SpringLayout.WEST, editStudentBtn); layout.putConstraint(SpringLayout.NORTH, clearBtn, 240, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, clearBtn, 80, SpringLayout.WEST, deleteStudentBtn); layout.putConstraint(SpringLayout.NORTH, deleteStudentBtn, 240, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, sortStudentGPABtn, 300, SpringLayout.WEST, panel); layout.putConstraint(SpringLayout.NORTH, sortStudentGPABtn, 330, SpringLayout.NORTH, panel); layout.putConstraint(SpringLayout.WEST, sortStudentNameBtn, 115, SpringLayout.WEST, sortStudentGPABtn); layout.putConstraint(SpringLayout.NORTH, sortStudentNameBtn, 330, SpringLayout.NORTH, panel); this.add(panel); this.pack(); this.setTitle("Student Information"); this.setSize(800, 420); // disable Edit and Delete buttons editStudentBtn.setEnabled(false); deleteStudentBtn.setEnabled(false); // enable Add button addStudentBtn.setEnabled(true); } public void showMessage(String message) { JOptionPane.showMessageDialog(this, message); } /** * hiển thị list student vào bảng studentTable * * @param list */ public void showListStudents(List<Student> list) { int size = list.size(); // với bảng studentTable có 5 cột, // khởi tạo mảng 2 chiều students, trong đó: // số hàng: là kích thước của list student // số cột: là 5 Object [][] students = new Object[size][5]; for (int i = 0; i < size; i++) { students[i][0] = list.get(i).getId(); students[i][1] = list.get(i).getName(); students[i][2] = list.get(i).getAge(); students[i][3] = list.get(i).getAddress(); students[i][4] = list.get(i).getGpa(); } studentTable.setModel(new DefaultTableModel(students, columnNames)); } /** * điền thông tin của hàng được chọn từ bảng student * vào các trường tương ứng của student. */ public void fillStudentFromSelectedRow() { // lấy chỉ số của hàng được chọn int row = studentTable.getSelectedRow(); if (row >= 0) { idField.setText(studentTable.getModel().getValueAt(row, 0).toString()); nameField.setText(studentTable.getModel().getValueAt(row, 1).toString()); ageField.setText(studentTable.getModel().getValueAt(row, 2).toString()); addressTA.setText(studentTable.getModel().getValueAt(row, 3).toString()); gpaField.setText(studentTable.getModel().getValueAt(row, 4).toString()); // enable Edit and Delete buttons editStudentBtn.setEnabled(true); deleteStudentBtn.setEnabled(true); // disable Add button addStudentBtn.setEnabled(false); } } /** * xóa thông tin student */ public void clearStudentInfo() { idField.setText(""); nameField.setText(""); ageField.setText(""); addressTA.setText(""); gpaField.setText(""); // disable Edit and Delete buttons editStudentBtn.setEnabled(false); deleteStudentBtn.setEnabled(false); // enable Add button addStudentBtn.setEnabled(true); } /** * hiện thị thông tin student * * @param student */ public void showStudent(Student student) { idField.setText("" + student.getId()); nameField.setText(student.getName()); ageField.setText("" + student.getAge()); addressTA.setText(student.getAddress()); gpaField.setText("" + student.getGpa()); // enable Edit and Delete buttons editStudentBtn.setEnabled(true); deleteStudentBtn.setEnabled(true); // disable Add button addStudentBtn.setEnabled(false); } /** * lấy thông tin student * * @return */ public Student getStudentInfo() { // validate student if (!validateName() || !validateAge() || !validateAddress() || !validateGPA()) { return null; } try { Student student = new Student(); if (idField.getText() != null && !"".equals(idField.getText())) { student.setId(Integer.parseInt(idField.getText())); } student.setName(nameField.getText().trim()); student.setAge(Byte.parseByte(ageField.getText().trim())); student.setAddress(addressTA.getText().trim()); student.setGpa(Float.parseFloat(gpaField.getText().trim())); return student; } catch (Exception e) { showMessage(e.getMessage()); } return null; } private boolean validateName() { String name = nameField.getText(); if (name == null || "".equals(name.trim())) { nameField.requestFocus(); showMessage("Name không được trống."); return false; } return true; } private boolean validateAddress() { String address = addressTA.getText(); if (address == null || "".equals(address.trim())) { addressTA.requestFocus(); showMessage("Address không được trống."); return false; } return true; } private boolean validateAge() { try { Byte age = Byte.parseByte(ageField.getText().trim()); if (age < 0 || age > 100) { ageField.requestFocus(); showMessage("Age không hợp lệ, age nên trong khoảng 0 đến 100."); return false; } } catch (Exception e) { ageField.requestFocus(); showMessage("Age không hợp lệ!"); return false; } return true; } private boolean validateGPA() { try { Float gpa = Float.parseFloat(gpaField.getText().trim()); if (gpa < 0 || gpa > 10) { gpaField.requestFocus(); showMessage("GPA không hợp lệ, gpa nên trong khoảng 0 đến 10."); return false; } } catch (Exception e) { gpaField.requestFocus(); showMessage("GPA không hợp lệ!"); return false; } return true; } public void actionPerformed(ActionEvent e) { } public void valueChanged(ListSelectionEvent e) { } public void addAddStudentListener(ActionListener listener) { addStudentBtn.addActionListener(listener); } public void addEdiStudentListener(ActionListener listener) { editStudentBtn.addActionListener(listener); } public void addDeleteStudentListener(ActionListener listener) { deleteStudentBtn.addActionListener(listener); } public void addClearListener(ActionListener listener) { clearBtn.addActionListener(listener); } public void addSortStudentGPAListener(ActionListener listener) { sortStudentGPABtn.addActionListener(listener); } public void addSortStudentNameListener(ActionListener listener) { sortStudentNameBtn.addActionListener(listener); } public void addListStudentSelectionListener(ListSelectionListener listener) { studentTable.getSelectionModel().addListSelectionListener(listener); } }
5. Tạo lớp StudentController.java
File: StudentController.java
package vn.viettuts.qlsv.controller; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.List; import javax.swing.event.ListSelectionEvent; import javax.swing.event.ListSelectionListener; import vn.viettuts.qlsv.dao.StudentDao; import vn.viettuts.qlsv.entity.Student; import vn.viettuts.qlsv.view.StudentView; public class StudentController { private StudentDao studentDao; private StudentView studentView; public StudentController(StudentView view) { this.studentView = view; studentDao = new StudentDao(); view.addAddStudentListener(new AddStudentListener()); view.addEdiStudentListener(new EditStudentListener()); view.addDeleteStudentListener(new DeleteStudentListener()); view.addClearListener(new ClearStudentListener()); view.addSortStudentGPAListener(new SortStudentGPAListener()); view.addSortStudentNameListener(new SortStudentNameListener()); view.addListStudentSelectionListener(new ListStudentSelectionListener()); } public void showStudentView() { List<Student> studentList = studentDao.getListStudents(); studentView.setVisible(true); studentView.showListStudents(studentList); } /** * Lớp AddStudentListener * chứa cài đặt cho sự kiện click button "Add" * * @author viettuts.vn */ class AddStudentListener implements ActionListener { public void actionPerformed(ActionEvent e) { Student student = studentView.getStudentInfo(); if (student != null) { studentDao.add(student); studentView.showStudent(student); studentView.showListStudents(studentDao.getListStudents()); studentView.showMessage("Thêm thành công!"); } } } /** * Lớp EditStudentListener * chứa cài đặt cho sự kiện click button "Edit" * * @author viettuts.vn */ class EditStudentListener implements ActionListener { public void actionPerformed(ActionEvent e) { Student student = studentView.getStudentInfo(); if (student != null) { studentDao.edit(student); studentView.showStudent(student); studentView.showListStudents(studentDao.getListStudents()); studentView.showMessage("Cập nhật thành công!"); } } } /** * Lớp DeleteStudentListener * chứa cài đặt cho sự kiện click button "Delete" * * @author viettuts.vn */ class DeleteStudentListener implements ActionListener { public void actionPerformed(ActionEvent e) { Student student = studentView.getStudentInfo(); if (student != null) { studentDao.delete(student); studentView.clearStudentInfo(); studentView.showListStudents(studentDao.getListStudents()); studentView.showMessage("Xóa thành công!"); } } } /** * Lớp ClearStudentListener * chứa cài đặt cho sự kiện click button "Clear" * * @author viettuts.vn */ class ClearStudentListener implements ActionListener { public void actionPerformed(ActionEvent e) { studentView.clearStudentInfo(); } } /** * Lớp SortStudentGPAListener * chứa cài đặt cho sự kiện click button "Sort By GPA" * * @author viettuts.vn */ class SortStudentGPAListener implements ActionListener { public void actionPerformed(ActionEvent e) { studentDao.sortStudentByGPA(); studentView.showListStudents(studentDao.getListStudents()); } } /** * Lớp SortStudentGPAListener * chứa cài đặt cho sự kiện click button "Sort By Name" * * @author viettuts.vn */ class SortStudentNameListener implements ActionListener { public void actionPerformed(ActionEvent e) { studentDao.sortStudentByName(); studentView.showListStudents(studentDao.getListStudents()); } } /** * Lớp ListStudentSelectionListener * chứa cài đặt cho sự kiện chọn student trong bảng student * * @author viettuts.vn */ class ListStudentSelectionListener implements ListSelectionListener { public void valueChanged(ListSelectionEvent e) { studentView.fillStudentFromSelectedRow(); } } }
6. Tạo lớp FileUtils.java
File: FileUtils.java
package vn.viettuts.qlsv.utils; import java.io.File; import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; import javax.xml.bind.Marshaller; import javax.xml.bind.Unmarshaller; public class FileUtils { /** * Chuyển đổi đối tượng object về định dạng XML * Sau đo lưu vào fileName * * @param fileName * @param object */ public static void writeXMLtoFile(String fileName, Object object) { try { // tạo đối tượng JAXB Context JAXBContext jaxbContext = JAXBContext.newInstance(object.getClass()); // Create đối tượng Marshaller Marshaller jaxbMarshaller = jaxbContext.createMarshaller(); // formating jaxbMarshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE); // lưu nội dung XML vào file File xmlFile = new File(fileName); jaxbMarshaller.marshal(object, xmlFile); } catch (JAXBException e) { e.printStackTrace(); } } /** * Đọc nội dung fileName, sau đó chuyển đổi nội dung của file * thành đối tượng có kiểu là clazz. * * @param fileName * @param clazz * @return */ public static Object readXMLFile(String fileName, Class<?> clazz) { try { File xmlFile = new File(fileName); JAXBContext jaxbContext = JAXBContext.newInstance(clazz); Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller(); return jaxbUnmarshaller.unmarshal(xmlFile); } catch (JAXBException e) { e.printStackTrace(); } return null; } }
III. Tạo lớp App.java
File: App.java
Lớp này chứa phương thức main() để chạy ứng dụng.
package vn.viettuts.qlsv; import java.awt.EventQueue; import vn.viettuts.qlsv.controller.LoginController; import vn.viettuts.qlsv.view.LoginView; public class App { public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { LoginView view = new LoginView(); LoginController controller = new LoginController(view); // hiển thị màn hình login controller.showLoginView(); } }); } }
Run bài tập quản lý sinh viên trong java swing
Demo
1. Login
Login với username/pasword: admin/admin:
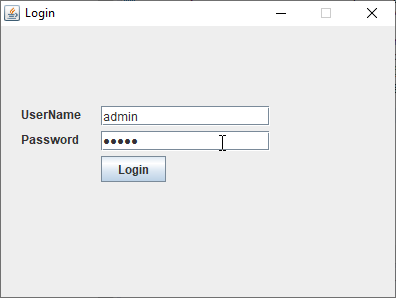
Màn hình quản lý sinh viên:
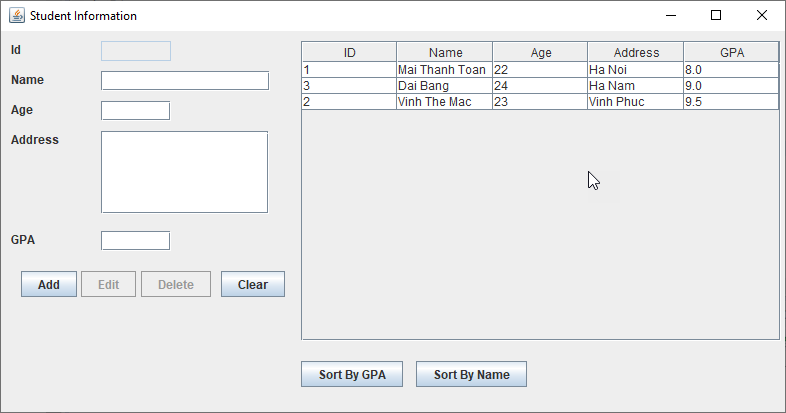
2. Thêm sinh viên
Nhập thông tin sinh viên:
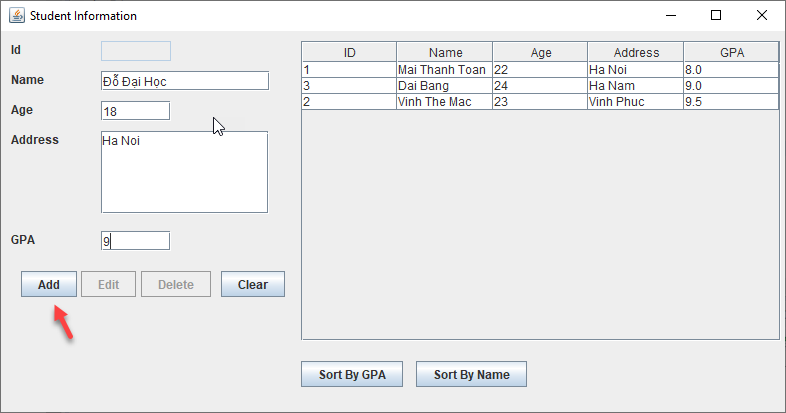
Click Add button:
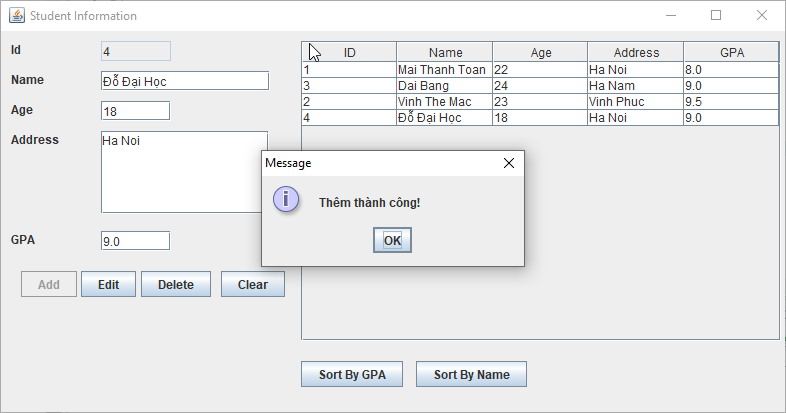